- Remote glassfish interaction
- Java 7 support
- Maven 3 support
- HTML 5 support
- JSON formatter
- Git natively integrated
- JUnit is now an unbundled plugin (Oracle legal had problems with the old CPL license)
- easy JPA 2 metamodel generation
18 March 2012
NetBeans 7 new features (edit)
NetBeans 7 is approved for release now. Here's a list of new features
15 March 2012
Java How To Program: alternate labs
Chapter 2
Preparation
- Make sure your PATH environment variable contains the JDK bin directory
- On windows separate PATH entries using ; (semicolon)
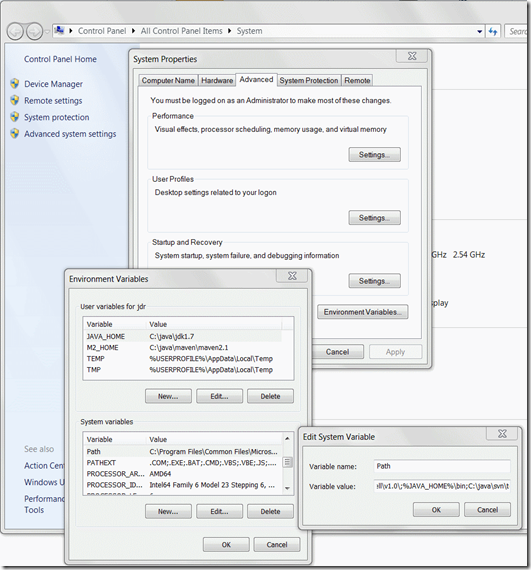
Body Mass Index Calculator
- Using an editor create a file called BmiTest.java
- Add a public class called BmiTest to the file
- Add a main method to the BmiTest class. In the main method
- Ask the user for his weight in grams
- Ask the user for his height in centimeters
- Print the Body Mass Index of the user, using the formula
BMI = weight x 10 / (height x height)
- Save the file
- Compile the file using the javac compiler
- Run the file using the java command
- Correct any errors.
- Compile, run and correct errors until all is well.
Chapter 3
Body Mass Index Calculator
- Create a new Java Application project called javase
- Consult the tutorial on creating a NetBeans Java project
- Do not create a main class
- Consult the tutorial on creating a NetBeans Java project
- In the project browser window, right click your project and select New => Java Class
- Call the class Bmi
- Add private double fields to the class called weight and height
- Add a constructor to the class
- constructor signature: public Bmi (double myWeight, double myHeight)
- In the constructor initialise the object fields using the constructor parameters
- Add a method that calculates the BMI
- Signature: public double calculate()
- In the method return the calculated bodymass. Adapt the calculation to use weight in kilogram and height in meters.
- Copy the BmiTest class to the project
- Modify the class to
- Request weight in kilogram and height in meters
- Accept floating point numbers
- Replace the calculation with
- Creation of a Bmi object called bmi
- Calculate the body mass index using the calculate() method on the bmi object
- In the main method, print out the result of the calculation
- Run the main method by clicking on the green arrow button in the top toolbar
- Correct any errors and run again until all is well.
Chapter 4
Body Mass Index Calculator
A normal BMI is between 18.5 and 24.9.- In the BMI class add a method with signature: public double deviation()
- The method should return 0.0 if the BMI is within the normal range
- The method should return a negative number indicating the difference with 18.5 if the BMI is lower
- The method should return a positive number indicating the difference with 24.9 if the BMI is higher
- In the BmiTest main class
- print a message indicating that the weight is normal if the difference with the bounds is less than 0.1
- otherwise print a message indicating how much the weight should change to be within the normal range
- create a main class
Chapter 5
Facebook Growth Prediction
In July 2010 facebook had 500 million users and was growing with a rate of 5% each month.Assume the growth continues at this pace.
Write a class called FacebookFuture with a main method that lists the number of users for each of the following months, until the number of users exceeds one billion. For each month print out a line with
- an incrementing number indicating how many months this is from the start date
- the month and year in a mm/yyyy format
- the number of users
Tip: since Java 7 you may use underscores in numbers to enhance readability. Example:
long billion=1_000_000_000;Info: At the beginning of 2012 facebook numbered 850.000 users
Chapter 6
Facebook Growth Prediction
To calculate the target amount you have after a number of months the formula istarget = (1 + rate)months X amountTo calculate the number of months it takes to the target amount, the formula is
log(target/amount)/log(1+rate)=months
- Create a class GrowthPrediction.
- Add an attribute for the current amount (double)
- Add a constructor that initializes the amount.
- Add a method with signature: public int growthCycles(double target, double rate).
- Create a test class called GrowthPredictionTest
- Right click the GrowthPrediction class and select Tools => Create JUnit Tests
- Accept all defaults
- Use JUnit 4
- In the testGrowthCycles method add a test that verifies the numbers you obtained in the previous excercise.
- Put the number of months obtained in the previous exercise in the expResult variable
- Remove the fail statement and the comment above it
- Print out a message saying how many months it would take for the number of facebook users to reach one billion
- Run the test using the top menu Run => test
- Using the same conditions add code to the test method to calculate how many months it would take for facebook to have as many users as a world population of 7_000_000_000
- Print out the result
- Right click the GrowthPrediction class and select Tools => Create JUnit Tests
Chapter 7
Game of Craps
- Make these modifications to the Craps code (Fig 6.9):
- Comment out all printing in the Craps class
- Make the number of rolls and the result attributes instead of local variables
- Add methods
- public int getRolls();
- public boolean getResult();
- Make these modifications to the CrapsTest class.
- Run the games for a number of times passed as a command like argument
- Run CrapsTest from the command line
- If you run in netbeans, in the project pane, right click the project => properties => run and set the command line arguments
- Print out how many games were won and lost on the first, second,… , twentieth roll and after the twentieth roll.
- Print out the percentage of games won
- Print out the average number of rolls in a game
- Run the games for a number of times passed as a command like argument
Chapter 8
Bank
Implement the Bank case study.- Put classes in two packages
- jhtp.bank
- jhtp.bank.atm
- Start with the classes at the right bottom of Fig 8.24 and work up
- Return dummy 0 equivalents from the methods to comply with the method return types
- Add attributes for the relations in Fig 8.25
Cards
- Add two new enum classes to example 7.09:
- Face
- Suit
- Modify the example to use the enums
- Replace the decksize (52) with a number calculated from the available enums
- Run the test program and correct errors until it succeeds
Chapter 9
Bank
- Make the SavingsAccount from exercise 8.6 a subclass of the Account from the ATM case study.
- Replace the usage of the savingsBalance with getter/setter methods using the totalBalance of the Account class.
- Add the necessary support to the Account class
- Remove the savingsBalance attribute
- Test the modified savingsBalance using the SavingsAccount test and correct errors until it succeeds
Javadoc
- Comment the bank classes, methods and attributes with javadoc
- In the project browser richt click on the project and select Generate javadoc
- Review the generated javadoc, adapt and regenerate
- Go to the files prowser tab and open the dist/javadoc directory where the javadoc is generated. Open the file stylesheet.css and append the contents of this file to it and save it. Reload the javadoc in another browser tab and check if anything changed in the presentation.
Chapter 10
Bank
- Add The Transaction class to the Bank exercise
CommissionEmployee
- Start from the BasePlusCommissionEmployee code in example fig09_12_14
- BasePlusCommissionEmployee inherits from CommissionEmployee. We will replace inheritance with composition.
- Rename CommissionEmployee to CommissionOnlyEmployee
- Create an interface CommissionEmployee that contains the public methods of CommissionOnlyEmployee (except toString())
- In CommissionOnlyEmployee choose Refactor => Extract Interface
- Adapt BasePlusCommissionEmployee to delegate to CommisionOnlyEmployee instead of inheriting from it
- Replace inheritance in BasePlusCommissionEmployee with inplementation of the CommissionEmployee interface.
- Add an attribute CommissionOnlyEmployee called delegate to BasePlusCommissionEmployee
- Instantiate the attribute in the constructor
- Replace all calls to super with calls to delegate
- implement all interface methods and call the corresponding method on delegate from them.
Chapter 13
Game Of Craps
Add exception handling to handle bad program parameters to the CrapsTest class in the Game Of Craps from Chapter 7.Handle two specific exceptions using one catch statement:
- no command line parameter was supplied
- the command line parameter was not an integer
Chapter 19
FileMatch 1: Collections
- Rewrite exercise 14.8, this time loading all accounts in a Collection in memory.
- Make sure the output is still ordered by account number.
FileMatch 2: ResourceBundles
Localise the messages in the application using resource bundles.- Pass a parameter to FileMatchTest indicating the language (fr or nl)
- In the main method construct a ResourceBundle with parameters
- “errormsg” as name for the properties files
- If the language was passed, a Locale for this language
- if no language was passed, use the one parameter constructor
- Pass the ResourceBundle to the FileMatch constructor and save it in an attribute
- Print errormessages using the ResourceBundle
- Use MessageFormat to substitute parameters in the message
- In the src directory create
- errormsg.properties (english)
- errormsg_fr.properties (french)
- errormsg_nl.properties (dutch)
- Add the keys used in the FileMatch error messages to all files and add translated messages in the three languages
Chapter 23
FileMatch
In this exercise we will process multiple transaction files concurrently.- Create a class bank.TransactionProcessor that implements Runnable
- Add a constructor TransactionProcessor(Map<Integer, Account> accounts, Path transactionFile)
- store the parameters in attributes.
- Implement the run method of TransactionProcessor. Move the code from FileMatch that reads the transaction file and adds the transaction amount to the corresponding accounts here. Adapt the code to use the instance attributes.
- Add a constructor TransactionProcessor(Map<Integer, Account> accounts, Path transactionFile)
- Make about 5 copies of the trans.txt file.
- Give them similar names so you can retrieve them using a wildcard.
- Adapt the FileMatch class
- Make the Account Collection synchronised to allow concurrent access.
- Read the oldmast.txt file and store all accounts in the Account Collection
- Create an ExecutorService cached trheadpool
- For each transaction file
- create a TransactionProcessor thread. Pass the Account collection and the file Path to it.
- execute the thread
- shutdown the threadpool and awaitTermination of all threads
- Write the updated Account Collection to the newmast.txt file.
- Add some System.out.println statements throughout your code to track the processing of the files
- Run the program and verify the results.
Chapter 30
FileMatch
Write a program that replaces the blank character separators in oldmast.txt with a colon (:) separator. A sequence of blank characters should be replaces with only one colon separator.13 March 2012
QR code shopping
Uniway adds QR codes to TV shopping program.
You can consult product info on your smartphone and get called by the call center to take your order.
Labels:
e-commerce,
QR code,
TV,
uniway
Subscribe to:
Posts (Atom)